How to Check if a Specific .NET Framework Version is Installed using Inno Setup
Many applications require additional components installed on the target system to run properly. Therefore, before installing an application, it’s important to ensure all prerequisites are met. One common prerequisite is the .NET Framework.
This tutorial will guide you through the process of creating an Inno Setup installer package that verifies if a specific .NET Framework version is installed on the target system.
It also introduces a simpler alternative with Advanced Installer. But first, let's walk through how to create the script using Inno Setup.
How to Create the Script using Inno Setup?
When creating an installer package with Inno Setup, you must define all the installer parameters through a script. These parameters include the application name, version, included files, or any action the installer performs.
When you open the Inno Setup compiler, a Welcome dialog will appear. This dialog offers two options for creating the script:
- Create a new empty script: You will have to define all the information about the installer.
- Use the Script Wizard: This option allows you to define the basic information about your installer through a user interface. This information will be included in the script once it is created.
For this tutorial, choose to create a new empty script.
How to Configure the General Settings of the Installer
Once you have the application, it’s time to write the script to configure the installer. The script is divided into several general sections and here are some of the common ones:
- Setup: Includes global settings used by the installer and uninstaller.
- Files: Specifies the files to be included in the installation package and their destination directory.
- Icons: Defines the shortcuts to be created in the Start Menu or other locations.
- Tasks: Defines the tasks that are performed during installation.
Here’s an example of what the script including these sections looks like:
[Setup] AppName=DemoApp AppVersion=1.0 DefaultDirName={pf}\DemoApp DefaultGroupName=Demo App OutputDir=C:\Users\R\Desktop\AppInstaller OutputBaseFilename=DemoApp_Setup Compression=lzma SolidCompression=yes PrivilegesRequired=admin [Files] Source: "C:\Users\R\Desktop\sample.exe"; DestDir: "{app}"; Flags: ignoreversion [Icons] Name: "{group}\DemoApp"; Filename: "{app}\sample.exe" Name: "{commondesktop}\DemoApp"; Filename: "{app}\sample.exe"; Tasks: desktopicon [Tasks] Name: "desktopicon"; Description: "Create a &desktop icon"; GroupDescription: "Additional icons:"; Flags: unchecked
Check for the .NET Framework
Once you’ve completed the script with the general sections, it’s time to add the Code section to check for the .NET Framework and prompt the user to install it if it is not present on the target machine.
The Code section allows the use of Pascal scripts to customize the setup. I’ve used two functions in this section:
- The first function queries the Windows Registry: This checks if a specific .NET Framework version is installed.
For .NET Framework 4.x, it’s enough to ensure the ‘Version’ key exists and starts with ‘4.’.
However, for a sub-version, you need to check the release key.
For example, to check if version 4.8.1 is installed, you have to check if the release version is 533330 or higher, corresponding to .NET Framework 4.8.1.
[Code] function IsDotNetFrameworkInstalled(): Boolean; var success: Boolean; releaseVersion: Cardinal; begin success := RegQueryDWordValue(HKLM, 'SOFTWARE\Microsoft\NET Framework Setup\NDP\v4\Full', 'Release', releaseVersion) or RegQueryDWordValue(HKLM, 'SOFTWARE\Microsoft\NET Framework Setup\NDP\v4\Client', 'Release', releaseVersion); Result := success and (releaseVersion >= 533320); end;
- The second function initializes the setup: This function controls the setup process, continuing or abording it depending on the .Net Framework installation.
It calls the first function to check for the .NET Framework 4.8.1 and displays a message informing the user about the status of the .NET Framework installation.
function InitializeSetup(): Boolean; begin if IsDotNetFrameworkInstalled() then begin MsgBox('.NET Framework 4.8.1 is installed on this system.', mbInformation, MB_OK); end else begin MsgBox('This application requires .NET Framework 4.8.1. Please install it before running this setup.', mbError, MB_OK); Result := False; Exit; end; Result := True;
All you have to do now is compile the script to create the installer and then run it to install the application.
If you want to check for .NET instead of .NET Framework, simply change the registry path and eliminate the release key check.
[Code] function IsDotNetVersionInstalled(const Version: String): Boolean; var InstalledVersion: String; begin Result := False; if RegQueryStringValue(HKEY_LOCAL_MACHINE, 'SOFTWARE\WOW6432Node\dotnet\Setup\InstalledVersions\x64\hostfxr', 'Version', InstalledVersion) then begin if InstalledVersion = Version then begin Result := True; end; end; end; procedure InitializeWizard; begin if not IsDotNetVersionInstalled('7.0.20') then begin MsgBox('This application requires .NET 7.0.20 to be installed. Please install the required .NET version and try again.', mbError, MB_OK); Abort(); end; end;
Now that you know how to verify .NET Framework installation with Inno Setup, let’s take a look at how to do that using Advanced Installer.
How to Check if .NET Framework is Installed Using Advanced Installer
Advance Installer is a powerful tool for installing, updating, and configuring your product. It provides an easy-to-use GUI to help you master your installer package.
Check out this article to learn how to create an installer package in Advanced Installer.
Once you’ve created a package project in Advanced Installer, follow these steps to verify if the .NET Framework is installed on the target system:
1. Navigate to the Launch Conditions page and access the Software tab.
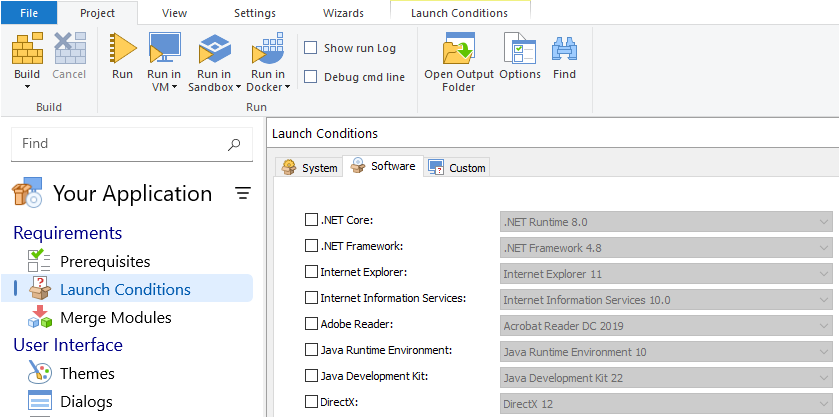
2. Check the .NET Framework option and then select the specific version that your application requires. The package will be installed only if the selected version or higher one is found on the machine.

If you need to check for other components, select them from the list along with the specific version. If the components you need are not listed, open the Custom section to define custom launch conditions using installer properties or environment variables.
Now, you only need to build the project to generate the MSI and install the application.
Conclusion
Using Inno Setup, you can restrict the software installation if a specific component that your software relies on is not installed on the target system.
However, Inno Setup requires defining scripts to set installer parameters and actions, which can be complex and time-consuming. On the other hand, Advanced Installer simplifies and accelerates the process with its user-friendly interface and more advanced capabilities.
Advanced Installer offers a 30-day full-feature trial. Give it a try today.