PowerShell & Intune, Automate your Work - Part 5: Check for Win32 App Relationships
Hello again! Let's dive into the next installment of our series where we simplify your workload using PowerShell and Intune.
Today, we'll explore how to check if a particular Win32 application in Intune has any dependencies or is superseded by other applications.
Before we jump into the fresh material, let’s quickly recap what we've covered so far.
Recap of Parts 1, 2, 3, and 4
We started our journey with the basics of using the AZ Module and how to authenticate with Azure, then moved on to harnessing the power of Microsoft Graph through PowerShell.
In the previous parts, we learned how to retrieve all Win32 apps, their assignments, dependencies, and even how to figure out which apps are superseded by others in Intune.
This has set a solid foundation for us to now delve into examining relationships between these applications.
Check for Dependencies and Supersedence
When managing applications in an enterprise environment, knowing the relationships between applications can be crucial.
Dependencies need to be installed for an app to function correctly, whereas superseding an app means replacing an older version with a new one. This makes sure everyone is up-to-date without cluttering systems with outdated software.
To start checking these relationships, we need to first connect to Microsoft Graph with the appropriate permissions. This is handled by:
Connect-MgGraph -Scopes "User.Read.All", "Group.ReadWrite.All", "DeviceManagementApps.Read.All", "DeviceManagementApps.ReadWrite.All", "GroupMember.Read.All", "Directory.Read.All", "Directory.ReadWrite.All", "Group.Read.All"
This line establishes a connection with broad permissions across user data and device management, ensuring we can access and manipulate the Win32 app data.
Once connected, we fetch the list of Win32 mobile apps using:
$Win32MobileApps = Invoke-MgGraphRequest -Method GET "https://graph.microsoft.com/v1.0/deviceAppManagement/mobileApps?$filter=isof('microsoft.graph.win32LobApp')"
This command specifically retrieves all Win32 Line of Business (LOB) apps managed in Intune.
Afterwards, if we combine what we learned in PowerShell & Intune, Automate your Work - Part 4: Retrieve Win32 Superseding Apps and PowerShell & Intune, Automate your Work - Part 3: Manage Win32 App Dependencies we can output the following script:
Connect-MgGraph -Scopes "User.Read.All", "Group.ReadWrite.All", "DeviceManagementApps.Read.All", "DeviceManagementApps.ReadWrite.All", "GroupMember.Read.All", "Directory.Read.All", "Directory.ReadWrite.All", "Group.Read.All" $Win32AppList = New-Object -TypeName "System.Collections.Generic.List[Object]" $Win32AppAssignmentList = New-Object -TypeName "System.Collections.Generic.List[Object]" $Win32MobileApps = Invoke-MgGraphRequest -Method GET "https://graph.microsoft.com/v1.0/deviceAppManagement/mobileApps?`$filter=isof('microsoft.graph.win32LobApp')" $RelationshipExistence = $false $type = "Dependency" if ($Win32MobileApps -ne "") { $Win32MobileApps = $Win32MobileApps.value if ($Win32MobileApps -ne $null) { foreach ($Win32MobileApp in $Win32MobileApps) { $Win32MobileApps2 = Invoke-MgGraphRequest -Method GET "https://graph.microsoft.com/beta/deviceAppManagement/mobileApps/$($Win32MobileApp.id)/relationships" #$Win32AppAssignmentMatches = $Win32MobileApps2.value | Where-Object { ($PSItem.target.'@odata.type' -like "*groupAssignmentTarget") -or ($PSItem.intent -like $Intent) } if ($Win32MobileApps2.value -ne $null) { switch ($Type) { "Dependency" { if ($Win32MobileApps2.value.'@odata.type' -like "#microsoft.graph.mobileAppDependency") { $RelationshipExistence = $true } } "Supersedence" { if ($Win32MobileApps2.value.'@odata.type' -like "#microsoft.graph.mobileAppSupersedence") { $RelationshipExistence = $true } } } } return $RelationshipExistence } }}
If you have a look in the code, you’ll see this line:
$type = "Dependency"
That particular variable can be either “Dependency” or “Supersedence”. What this script does at a basic level is search all the apps inside your tenant and mention if there are any dependencies or supersedences available in your apps as they are configured.
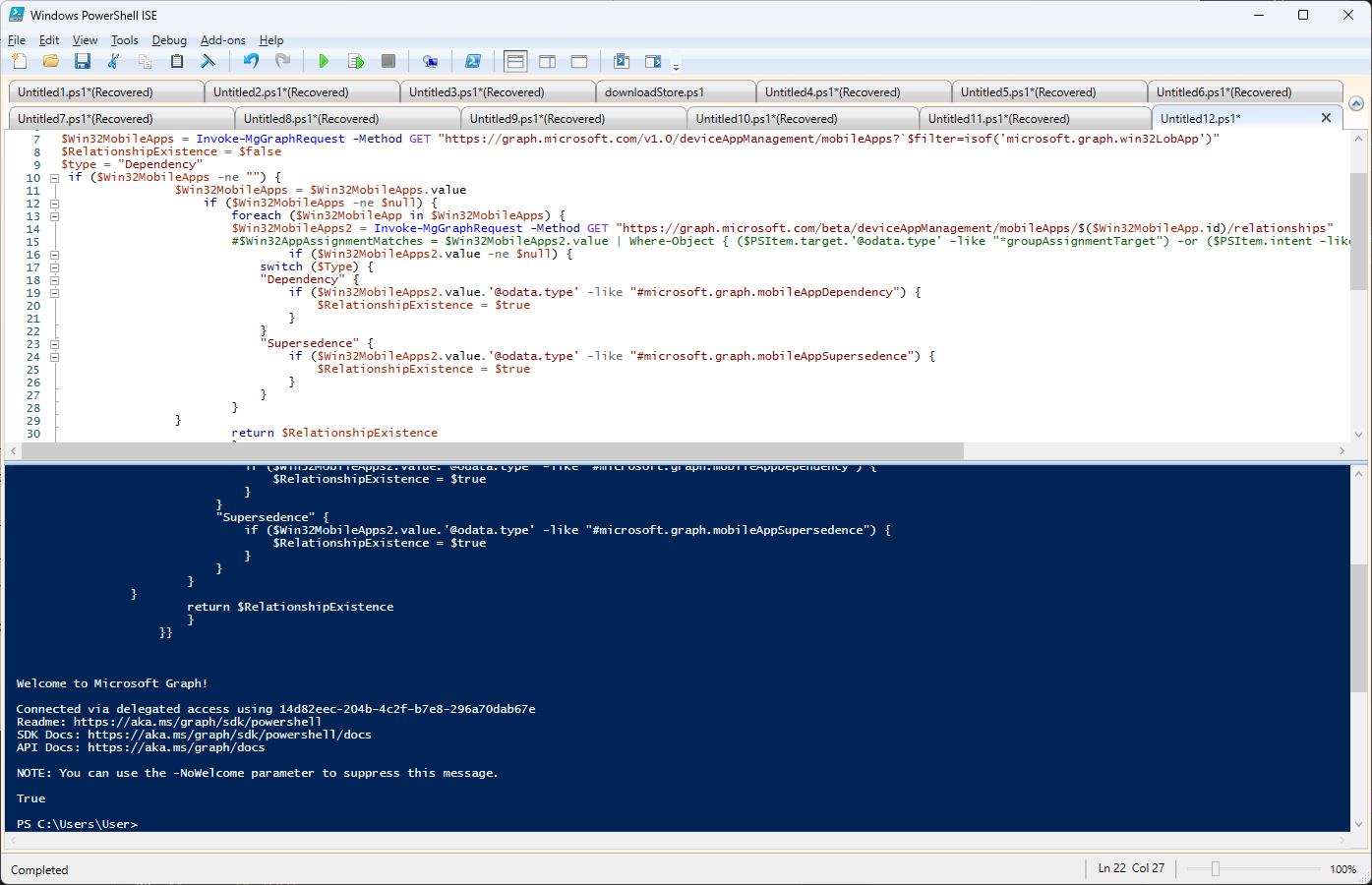
Check for Dependencies and Supersedence for a Particular App
While the above code might not be very useful because it doesn't output which application contains a dependency or supersedes another application, let's zoom into a more helpful scenario where we check if a particular app has any dependencies or supersedence.
The real magic happens when we iterate through these apps to check for any dependencies or superseding relationships.
Here’s a snippet where we analyze these relationships:
if ($Win32MobileApps -ne "") { $Win32MobileApps = $Win32MobileApps.value if ($Win32MobileApps -ne $null) { foreach ($Win32MobileApp in $Win32MobileApps) { if($Win32MobileApp.displayName -like "*7*"){ $Win32MobileApps2 = Invoke-MgGraphRequest -Method GET "https://graph.microsoft.com/beta/deviceAppManagement/mobileApps/$($Win32MobileApp.id)/relationships" if ($Win32MobileApps2.value -ne $null) { switch ($Type) { "Dependency" { if ($Win32MobileApps2.value.'@odata.type' -like "#microsoft.graph.mobileAppDependency") { $RelationshipExistence = $true } } "Supersedence" { if ($Win32MobileApps2.value.'@odata.type' -like "#microsoft.graph.mobileAppSupersedence") { $RelationshipExistence = $true } } } } } return $RelationshipExistence } } }
This script filters for apps whose names include a "7" (in our case we were checking for 7zip), retrieves their relationships, and checks if those relationships are either dependencies or supersessions based on their type. We switch between checking for dependencies and superseding relationships using the `$Type` variable.
Understanding these relationships helps in managing the lifecycle of applications more efficiently, ensuring that dependencies are respected and only the latest versions are deployed.
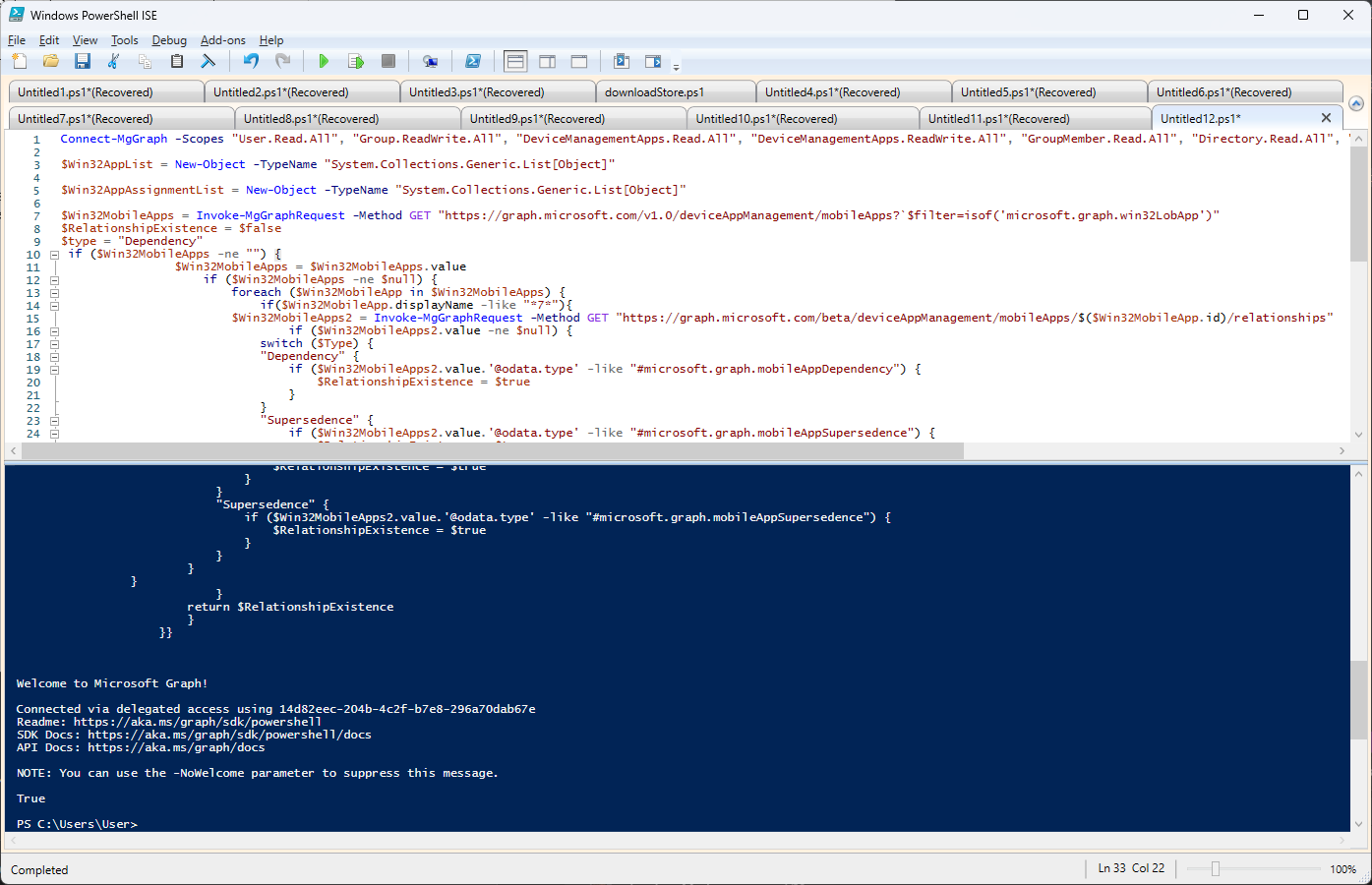
The full code for this is:
Connect-MgGraph -Scopes "User.Read.All", "Group.ReadWrite.All", "DeviceManagementApps.Read.All", "DeviceManagementApps.ReadWrite.All", "GroupMember.Read.All", "Directory.Read.All", "Directory.ReadWrite.All", "Group.Read.All" $Win32AppList = New-Object -TypeName "System.Collections.Generic.List[Object]" $Win32AppAssignmentList = New-Object -TypeName "System.Collections.Generic.List[Object]" $Win32MobileApps = Invoke-MgGraphRequest -Method GET "https://graph.microsoft.com/v1.0/deviceAppManagement/mobileApps?`$filter=isof('microsoft.graph.win32LobApp')" $RelationshipExistence = $false $type = "Dependency" if ($Win32MobileApps -ne "") { $Win32MobileApps = $Win32MobileApps.value if ($Win32MobileApps -ne $null) { foreach ($Win32MobileApp in $Win32MobileApps) { if($Win32MobileApp.displayName -like "*7*"){ $Win32MobileApps2 = Invoke-MgGraphRequest -Method GET "https://graph.microsoft.com/beta/deviceAppManagement/mobileApps/$($Win32MobileApp.id)/relationships" if ($Win32MobileApps2.value -ne $null) { switch ($Type) { "Dependency" { if ($Win32MobileApps2.value.'@odata.type' -like "#microsoft.graph.mobileAppDependency") { $RelationshipExistence = $true } } "Supersedence" { if ($Win32MobileApps2.value.'@odata.type' -like "#microsoft.graph.mobileAppSupersedence") { $RelationshipExistence = $true } } } } } return $RelationshipExistence } }}
Conclusion
As we continue to navigate through the complexities of application management in Intune using PowerShell, understanding the relationships between applications—specifically dependencies and supersessions—becomes essential.
Today, we've extended our toolkit by learning how to identify and verify these relationships for both broad sets of applications and individual apps. This capability not only enhances our management efficiency but also ensures that our application environments are streamlined and up-to-date.
With each part of this series, we're building a robust framework for automating tasks in Intune, making our IT administration tasks less daunting and more manageable.
Stay tuned for our next chapter, where we’ll dive even deeper into automation and discover new ways to leverage technology to our advantage.
Until then, keep exploring and automating!